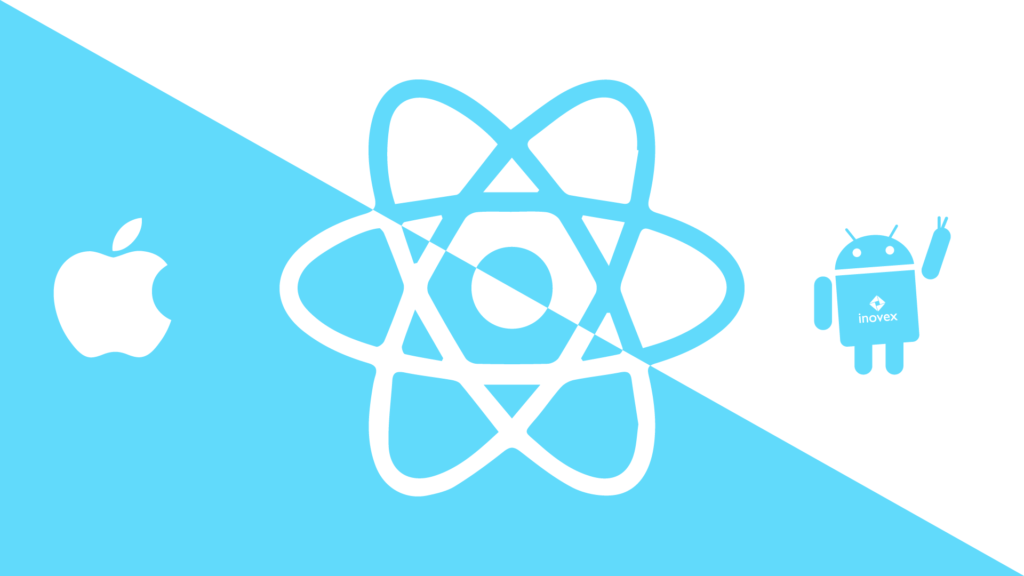
What is React?
React (also known as React.js or ReactJS) is open source JavaScript that provides an overview of data written in HTML. React previews are usually provided using components that contain additional components defined as custom HTML tags. React provides the developer with a model in which subcomponents cannot directly affect external components, efficiently updating the HTML document when changing data, and clearly separating components in today’s single-page applications.
ReactJS originated as a JavaScript port for XHP, a version of PHP that Facebook released four years ago. XHP is a PHP extension that increases the syntax of a language so that fragments of an XML document become valid PHP expressions. This allows you to use PHP as a more stringent template mechanism and offers a much simpler implementation of reusable components. XHP was primarily concerned with minimizing attacks on Cross-site Scripting (XSS). XSS attacks are facilitated when a malicious user enters content that aims to harm the viewer of that content. Typical vectors of attack are to enter content with embedded and hidden JavaScript (a language that works in every web browser), and then embedded JavaScript is used to steal information or otherwise compromise the user who views the content. XHP also reduces the worry of deleting information provided by users.
But there was a special problem with XHP because dynamic web applications require a lot of return travel to the server, and XHP did not provide solutions to this problem. In summary, the Facebook engineer negotiated with his manager to enter XHP into a browser using JavaScript and was given six months to try it out. The result was ReactJS.
Using React.js
If you pay attention to the development of JavaScript, you will know that “manipulating the DOM is expensive” has become a universal mantra. In other words, retrieving your JavaScript application data and “displaying” it in a browser is an expensive operation. It is therefore surprising that ReactJS is the first framework that makes this claim obvious. Optimizing JS for DOM manipulation leads to a fast library, in this case a library that allows the development of web applications that require very little code. ReactJS works by internally saving the state of your application and re-displaying your content in the browser (DOM manipulation), but only when the state changes. Namely, you interact with ReactJS by telling it when the state has changed and ReactJS manages all the visual changes of your application for you. This abstraction is useful and brilliant. The implementation is similar to AngularJS which manages DOM manipulation for you via two-way data connection, but ReactJS goes a step further because it knows when things have changed and when they haven’t, which makes a huge difference when building large applications. AngularJS relies on a dirty check and a tight loop, and my personal experience is that the AngularJS interface does not have the ability to be so intelligent in determining when a DOM change is unnecessary and to skip unwanted DOM manipulation. For large applications, I believe this makes a huge difference.
If you’re a computer expert, you’ll be fascinated by the way ReactJS documents the steps to building an efficient “differentiation” algorithm, an algorithm that understands when the state tree has changed. Namely, the initial approaches have O(n3) algorithmic complexity. Anyone who has ever been interviewed for the position of computer engineer knows that this is a set algorithm that should be avoided when working with anything other than small data sets. We can state that with a large web application with 10,000 DOM nodes, this would mean that it would take 17 minutes on a 1 GHz CPU to detect the difference in the trees. As Facebook engineers continued to work on ReactJS, they were able to optimize the algorithm to first achieve O(n2) complexity, which is a huge improvement, but then they were able to achieve O(n) complexity by using a hash map (HashMap) of DOM elements which contains unique keys. This was also a fascinating entry point into the true foundations of CS on which this wonderful library is based.
Knowing the steps used to achieve the specified algorithmic complexity helps explain why this library has a specific interface. When determining what to display, ReactJS relies on the “batch” step and the “pruning” step. These steps, for which your application can offer hints, suggest an understanding of the algorithm that operates behind the scenes. There is a learning curve with ReactJS in which you usually have to exchange data back and forth between the views and models that Facebook has made for it to process for you (AngularJS has revolutionized this step). The API for designated functions in ReactJS makes more sense when it is understood why it asks you to give hints at the time this exchange takes place.
Basically, the ReactJS interface is different from other JS libraries that are more imperative, which means you tell them directly when to change the DOM. For example, jQuery works that way. ReactJS is more like: here is my condition, and here is how you should interpret my condition and how it will change. So we can sit back and let ReactJS deal with the costly and complicated task of making a new condition visible to a user in a browser.
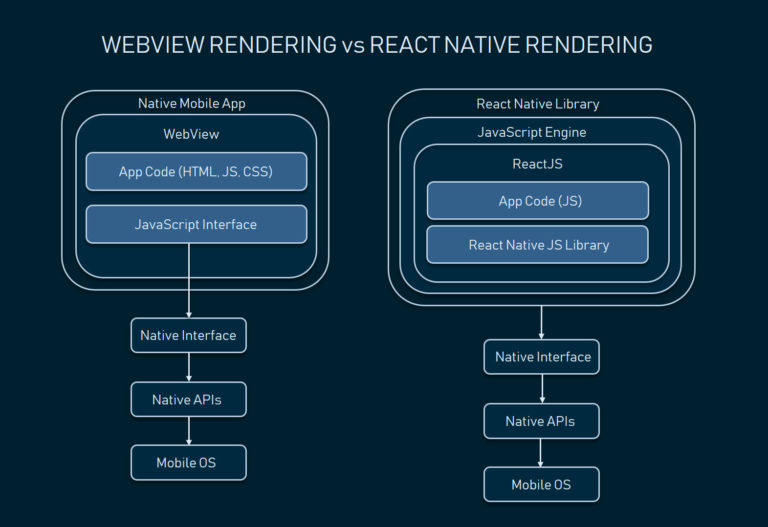
Try React
React is designed from the ground up for gradual adoption, and you can use as little or as much React as you need. Whether you want to try React, add a little interactivity to a simple HTML page, or run a complex application that runs React, the comments in this section will help you get started.
If you are interested in playing with React, you can use the various codes available online. Try the Hello World template on CodePen, CodeSandbox or Stackblitz.If you prefer to use your own text editor, you can download this HTML file, edit it and open it from the local file system in your browser. Due to the slow transformation of code during execution, it is recommended that this be used only for simpler demonstrations.
As your application grows, you can consider more integrated settings. There are several JavaScript tools we recommend for larger applications. Each of them can work with little or no configuration and allows you to take full advantage of the rich React ecosystem. This will be discussed in a future post.
React component
Of the structural things related to ReactJS, it remains only to explain what a component is, what its basic types are and how it can be used.
The React component is one of the basic building blocks of React applications. Each application you develop in React will be made up of parts called components. Components greatly facilitate the task of building a user interface.
Each component returns / renders some JSX code and defines which HTML code React should display in the right DOM at the end. JSX is not HTML, but it is very similar to it. In React, we generally have two types of components: functional components and class components.
– Functional components:
Simply put, functional components are JavaScript functions. By writing a JavaScript function we can create a functional component in React Apps. To make the application effective, we use a functional component only when we are sure that our component does not require interaction with any other component. Functional components do not require data from other components. Here is an example of a functional component in React:
function Title() { return <h1>I am Title</h1>; }
– Class components:
Class components are similar to a functional component, but have some additional features that make a class component a little more complex than functional components. Functional components do not care about other components in your application, while class components can work with each other. We can transfer data from one class component to another class component. Example of a class component in React:
class Title extends React.Component { render(){ return I am Title; } }
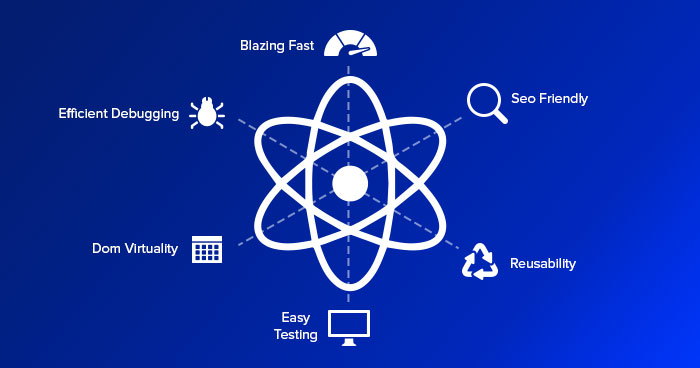
Conclusion
React makes the creation of interactive user interfaces painless. Design simple displays for each state in your application, and React will efficiently update and display the appropriate components when your data changes. Declarative representations in React make your code more predictable and easier to debug.
React allows you to connect to other libraries and frames. It can also render on the server using Node, or run mobile applications using React Native.
Enjoy React!